Effortless Client-Side Storage: Using IndexedDB with React Hooks
π¨π»π» Hello Devs,
Are you curious about client-side storage options? Today, let’s dive into a powerful tool called IndexedDB. If you’re a beginner in web development, you might wonder, “Is it a conventional database? Do we need to write queries? Can it replace the back-end?” Hold on — let’s clarify things first.
IndexedDB is a fully functional database available on the client side (browser). However, it is not meant to replace the back-end. So, relax and let’s explore more about it below.
Understanding the need or purpose of a tool is essential before learning about it. Let’s discuss a popular use case for IndexedDB. Imagine you’re building a chat application. Users will be texting and sharing messages through a server using APIs or Sockets while online. But what about accessing previous messages and chat data when offline? This is where IndexedDB comes into play.
In many popular chat applications, we can access profile data and previous messages even when offline. This is possible because the data is pre-stored in IndexedDB while the user is online and allowing access later, even without an internet connection.
π€ What is IndexedDB ?
Now that we know why we need it, let’s discuss what it is ?
In simpler terms, IndexedDB is a storage facility available on the client side (browser) that stores large amounts of structured data, making it easily accessible. The data can be of any type, whether primitive or non-primitive. IndexedDB is like an SQL-based Relational Database Management System (RDBMS). However, unlike SQL-based RDBMSes, which use fixed-column tables, It is a JavaScript-based object-oriented database. It lets us store and retrieve objects that are indexed with a key, any objects supported by the structured clone algorithm can be stored.
π Features provided by IndexedDB
To fully utilize any tool, we must understand its features. IndexedDB is easy and convenient to use if we understand its features and operations. Unlike other client-side storage options like local Storage, It supports storing complex data types, including objects, arrays, files, and blobs.
✔️ Its operations are transactional, ensuring data integrity. All read/write operations are part of a transaction, which is either completed successfully or rolled back in case of an error.
✔️ It is completely Asynchronous in nature, which means it never blocks the main thread and let us maintain the responsive user interface.
✔️ It allows creating indexes to enable efficient searching and querying of the data.
✔️ Data is stored in key-value pairs, where each data entry is uniquely identified by a key.
✔️ Its transactions are ACID-compliant, ensuring that operations are Atomic, Consistent, Isolated, and Durable.
✔️ Databases in IndexedDB are versioned. Each change to the database schema requires a new version, allowing for controlled upgrades and schema management.
✔️ It uses an event-driven model, where operations are initiated by dispatching events and then handling the corresponding event callbacks.
✔️ It is supported by all modern browsers, ensuring wide compatibility for web applications.
These features make IndexedDB a versatile and robust solution for client-side data storage in web applications.
π― Finally how to use IndexedDB ?
We can find many code snippets or examples for using IndexedDB, but what if I told you that we can manage the entire IndexedDB with a ReactJS hook? Yes, it is possible. We can manage the entire IndexedDB and all its operations by creating a simple custom ReactJS hook.
You can find a working demonstration of IndexedDB managed using a custom hook in this public GitHub Repository. This repository demonstrates how to use IndexedDB in a React application to manage and persist data locally. The project is created using Vite for a fast and optimized development environment. In this repository, I shared the simplest method I know. I hope that once you try it, you’ll also find it easy and precise.
For your understanding, I am explaining the code blocks step by step from the custom hook below.
Step 1. We have to open a connection with IndexedDB π΅
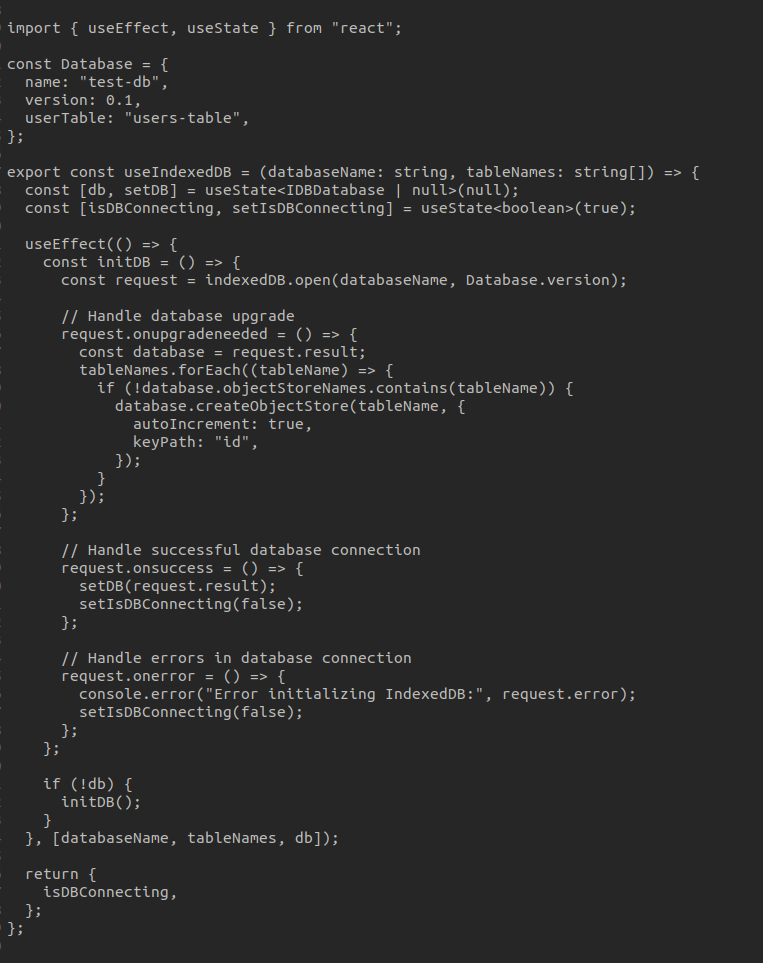
In the above example you can simply understand that we opened the connection with indexedDB, and named it “test-db” and provided version “0.1”.
It check if any instance of DB is already present with the given name and version then it connects with it and if not then it creates the new database with the given name and version,
Later on we initialised the request const with connection and, created the tables, while checking if the tables are not previously present, in case if the DB is connected to the existing DB
After successfully connecting with IndexedDB, you will be able to find the database and table in the dev tools of your browser in storage section of Application tabπ
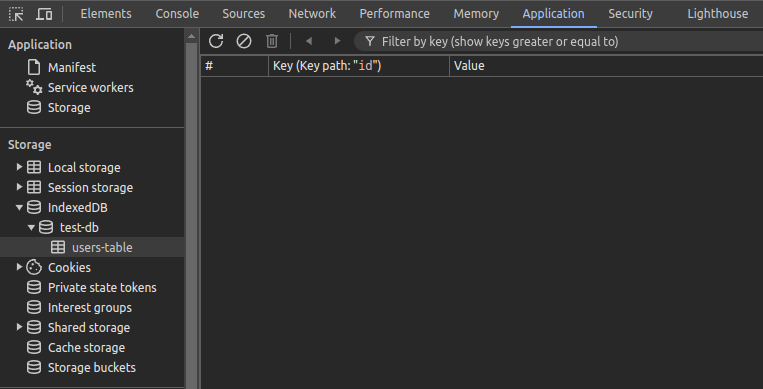
Step 2. Then we create a function to get transactionπ

Step 3. Then this is how we can insert or update a single indexed dataπ
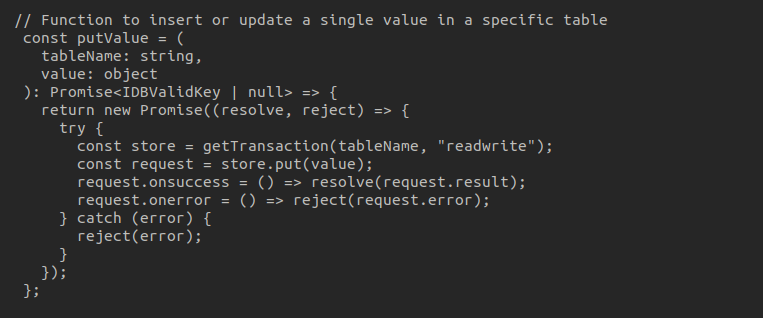
After adding the data in IndexedDB use the refresh button and than the record will look like thisπ
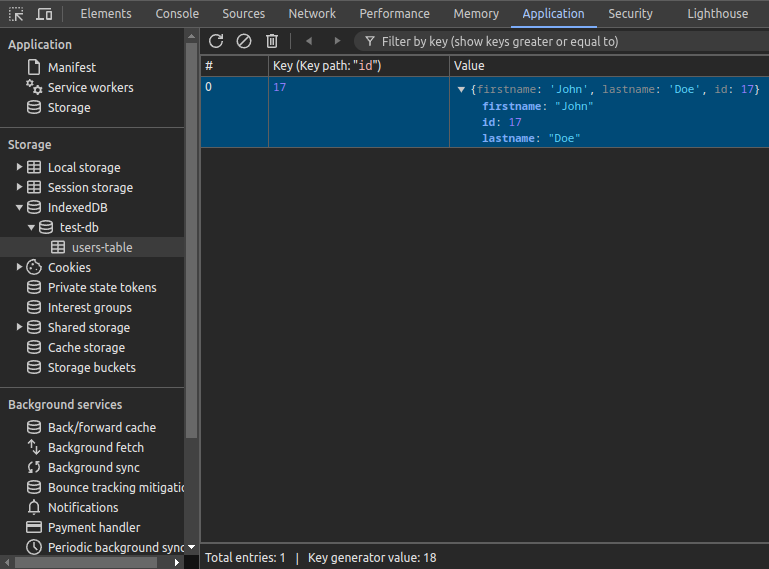
Step 4. This is how we can get dataπ
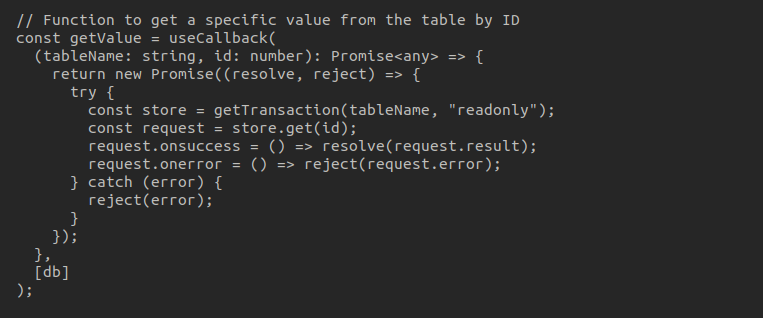
Step 5. This is how we can delete dataπ
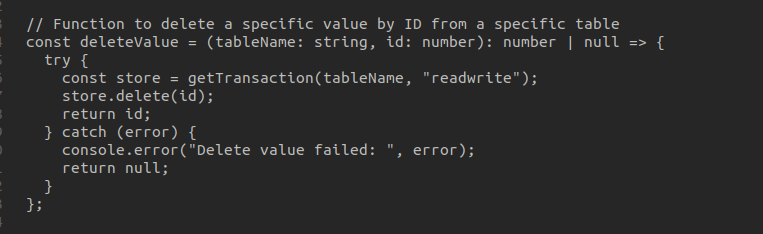
Similarly, I have also created methods for storing and retrieving bulk data in IndexedDB. The code blocks shared above are from a custom hook that I created to manage IndexedDB and all its operations. You can find all of this in this Github Repository.
π Summary
IndexedDB is a powerful client-side database that allows for efficient storage and retrieval of large amounts of structured data directly in the browser. By integrating IndexedDB with ReactJS through custom hooks, we can seamlessly manage database operations such as connecting, inserting, updating, and deleting data. This approach not only simplifies the code but also enhances the performance and responsiveness of our web applications. For a practical demonstration and to explore the full capabilities of IndexedDB check out the linked GitHub repository.
πVisit: https://github.com/manishgcodes/example-indexed-db
π€Happy coding!
Written by Manish Giri Goswami
Comments
Post a Comment